也许你已经了解了Spring的各种好处,但是自己往往很少亲手去搭建一个Spring的项目,本教程主要目的是通过简单的示例,来来搭一个Spring框架,体验Spring的好处。
工具准备
1:MyEclipse 10 + Jdk6.0 及以上
2:spring-framework-3.2.2.RELEASE-dist.zip + commons-logging-1.1.3.jar(Java日志实现)
构建环境
1:在MyEclipse里面新建一个工程,并命名称是Spring3test
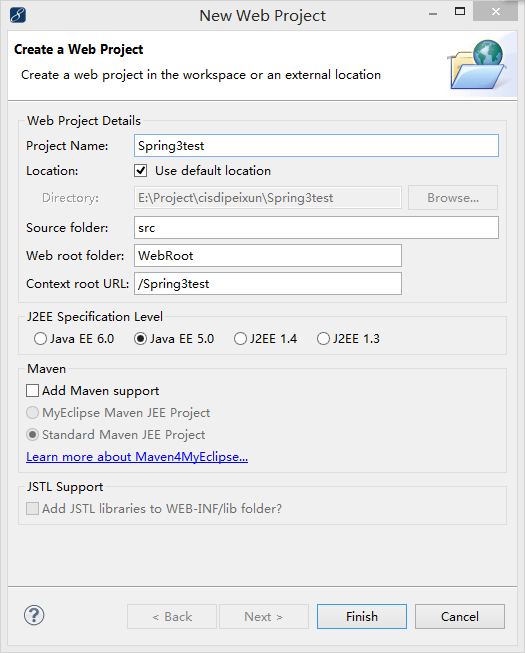
2:把发行包里面的lib下面的jar包都添加项目的lib文件夹下。我已经把所有的jar整理好了,点击下载
3:我们也可以根据Spring的工程来获取Spring需要的依赖包,在联网的情况下,通过Ant运行projects/build-spring-framework/build.xml,会自动去下载所需要的jar包,下载后的包位于projects/ivy-cache/repository下面。为了方便,把这些jar包也添加到MyEclipse里面。
配置文件
1: 编写接口IHelloApi.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.xhay1122.spring3.hello; /** * 项目名称:Spring3test * 类名称:HelloImpl * 类描述: * 创建人:xhay * 创建时间:2015-4-9 下午3:01:17 * 修改人:xhay * 修改时间:2015-4-9 下午3:01:17 * 修改备注: * @version 1.0 * 软件工程创新实验室 */ public interface IHelloApi { public String helloSpring3(String name); }
|
实现类HelloImpl.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.xhay1122.spring3.hello; /** * 项目名称:Spring3test * 类名称:HelloImpl * 类描述: * 创建人:xhay * 创建时间:2015-4-9 下午3:01:17 * 修改人:xhay * 修改时间:2015-4-9 下午3:01:17 * 修改备注: * @version 1.0 * 软件工程创新实验室 */ public class HelloImpl implements IHelloApi { public String helloSpring3(String name) { // TODO Auto-generated method stub System.out.println("Hello Spring3.------"+name); return "OK , name = "+name; } }
|
2:在src目录下面创建一个Spring的配置文件applicationContext.xml,内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <?xml version="1.0" encoding="utf-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd "> <!-- 用注解方式注入bean --> <context:annotation-config /> <!-- 装配bean --> <bean name="helloBean" class="com.xhay1122.spring3.hello.HelloImpl"></bean> </beans>
|
3: 编写测试代码HelloClient.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| /** * @Title: HelloClient.java * @Package com.xhay1122.spring3.test * @Description: TODO(用一句话描述该文件做什么) * @author matao@cqrainbowsoft.com * @date 2015-4-9 下午3:23:17 * @version V1.0 */ package com.xhay1122.spring3.test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.xhay1122.spring3.hello.IHelloApi; /** * 项目名称:Spring3test * 类名称:HelloClient * 类描述: * 创建人:xhay * 创建时间:2015-4-9 下午3:23:17 * 修改人:xhay * 修改时间:2015-4-9 下午3:23:17 * 修改备注: * @version 1.0 * 软件工程创新实验室 */ public class HelloClient { public static void main(String[] args) { // TODO Auto-generated method stub //获取ApplicationContext ApplicationContext ctx = new ClassPathXmlApplicationContext(new String[]{"applicationContext.xml"}); //从容器中获取hellBean(名字和applicationcontext.xml里面的id对应) IHelloApi api = (IHelloApi)ctx.getBean("helloBean"); String s = api.helloSpring3("xhay"); System.out.println("back word:"+s); } }
|
到此为止,简单的spring框架就配置好了:
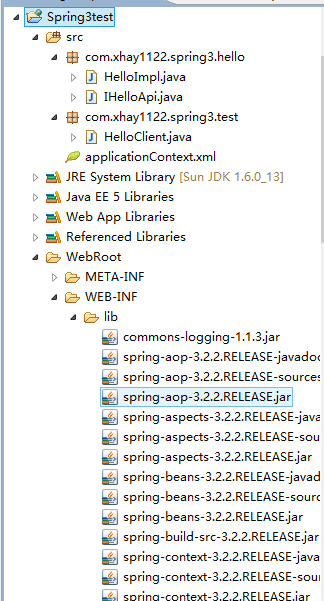
运行结果:
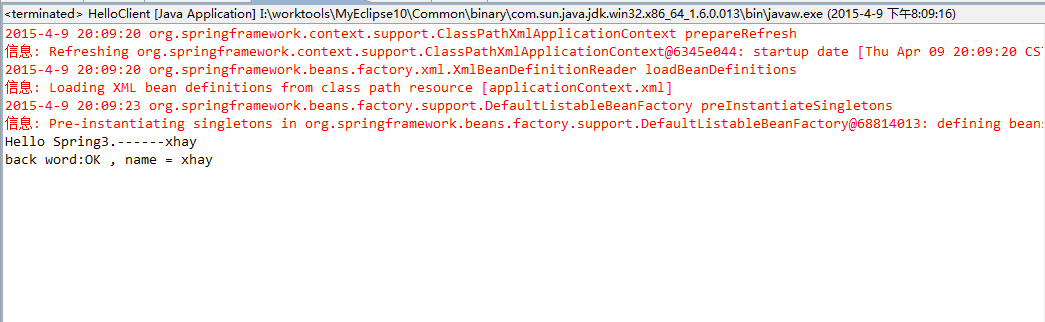
下载完整源代码